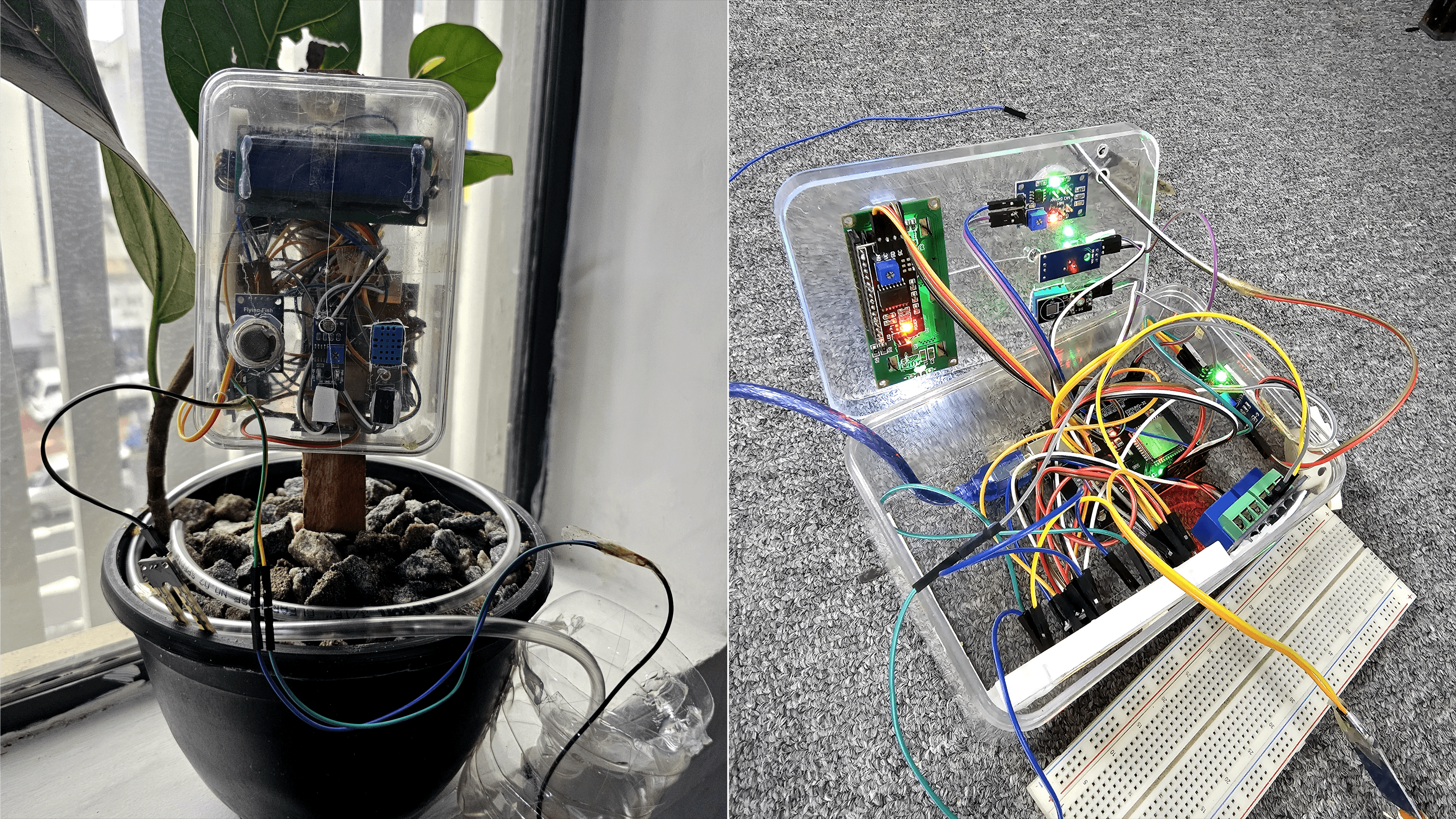
IoT-based Plant Monitoring System
Introduction
This project aims to develop an IoT-based system for monitoring various environmental parameters crucial for plant health, such as temperature, humidity, gas levels, and soil moisture. The system also includes an automatic watering feature that activates when the soil moisture level falls below a certain threshold. Additionally, users can manually override the automation via a user-friendly app.
Methodology
System Design and Architecture
The system consists of multiple sensors interfaced with a microcontroller to collect environmental data. An LCD display is used to show real-time data, while a relay module controls the water pump for automatic watering. The overall architecture integrates hardware and software components to ensure seamless operation and user control.
Hardware Components
Component | Description |
---|---|
DHT11 Sensor | for temperature and humidity measurement |
Soil Moisture Sensor | to monitor soil moisture levels |
LDR Module | for light detection |
MQ5 Gas Sensor | for gas level detection |
Relay Module | to control the water pump |
Buzzer | for alert signals |
LiquidCrystal I2C | for displaying data |
Software Components
The microcontroller is programmed to read data from the sensors and control the water pump and buzzer based on the readings. The program logic ensures automatic watering and alerts the user in case of gas detection. The user app allows manual control over the system.
Implementation
The implementation of the IoT-based plant monitoring system using the ESP32 Dev module involves integrating various sensors and components to collect environmental data and automate the watering process. Below is a detailed explanation of the implementation process, including code examples from the provided code.
Hardware Setup
Microcontroller (ESP32 Dev Module)
The ESP32 Dev module serves as the central unit that interfaces with the sensors and controls the relay and buzzer. The ESP32 offers built-in Wi-Fi and Bluetooth capabilities, making it suitable for IoT applications.
DHT11 Sensor
The DHT11 sensor measures temperature and humidity. It is connected to digital pin 32 on the ESP32.
Soil Moisture Sensor
The soil moisture sensor measures the moisture content in the soil. It is connected to analog pin 34. The sensor provides an analog value that is converted to a percentage to indicate soil moisture levels.
LDR (Light Dependent Resistor) Module
The LDR module detects light levels and is connected to digital pin 25. It helps determine whether the environment is dark or light.
MQ5 Gas Sensor
The MQ5 gas sensor detects the presence of gas and is connected to digital pin 33. It triggers an alert when gas is detected.
Relay Module
The relay module controls the water pump. It is connected to digital pin 23. When activated, it allows current to flow to the pump, turning it on or off based on the soil moisture level.
Buzzer
The buzzer is used to alert the user in case of gas detection. It is connected to digital pin 26.
LiquidCrystal I2C
An I2C-enabled LCD is used to display the sensor readings. It helps provide real-time feedback on temperature, humidity, soil moisture, and light levels.
Software Implementation
Library Inclusions: The program includes libraries for I2C communication, LCD control, and DHT sensor data processing:
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <DHT.h>
Pin Definitions: The pins connected to each sensor and component are defined at the beginning of the program. The soil moisture threshold is also defined to determine when to activate the water pump:
#define DHTPIN 32 // DHT11 sensor pin
#define DHTTYPE DHT11 // DHT 11
#define RELAY_PIN 23 // Relay pin for water pump
#define SOIL_MOISTURE_PIN 34 // Analog pin for soil moisture sensor
#define LDR_PIN 25 // Digital pin for LDR module
#define MQ5_PIN 33 // Digital pin for MQ5 gas sensor
#define BUZZER_PIN 26 // Pin for buzzer
const int threshold = 800; // Soil moisture threshold
Sensor and LCD Initialization: The DHT sensor and LCD are initialized, and pin modes are set in the setup()
function:
DHT dht(DHTPIN, DHTTYPE);
LiquidCrystal_I2C lcd(0x27, 16, 2); // Adjust the LCD address if needed
void setup() {
Serial.begin(9600);
pinMode(RELAY_PIN, OUTPUT);
digitalWrite(RELAY_PIN, LOW); // Ensure the relay is off initially
pinMode(LDR_PIN, INPUT);
pinMode(MQ5_PIN, INPUT);
pinMode(BUZZER_PIN, OUTPUT);
lcd.begin();
lcd.backlight();
dht.begin();
}
Sensor Data Reading and Processing: The loop()
function reads data from the sensors, processes it, and performs necessary actions:
void loop() {
// Read soil moisture
int soilMoistureValue = analogRead(SOIL_MOISTURE_PIN); // Read the value from the soil moisture sensor
int soilMoisturePercent = map(soilMoistureValue, 4095, 0, 0, 100); // Convert the value to a percentage
// Read DHT11 sensor
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
// Read LDR status
bool isDark = digitalRead(LDR_PIN) == LOW; // Assume LOW means dark, adjust if necessary
// Read MQ5 gas sensor
bool gasDetected = digitalRead(MQ5_PIN) == HIGH; // Adjust if necessary
}
Water Pump Control: The water pump is controlled based on the temperature and soil moisture readings. If the temperature exceeds 50 degrees Celsius or the soil moisture level falls below 20%, the relay activates to turn on the water pump:
// Control water pump based on temperature and soil moisture
if (temperature > 50 || soilMoisturePercent < 20) { // Temperature above 50 degrees or soil moisture below 20%
digitalWrite(RELAY_PIN, LOW); // Activate the relay (turn on the pump)
Serial.println("Pump ON");
} else {
digitalWrite(RELAY_PIN, HIGH); // Deactivate the relay (turn off the pump)
Serial.println("Pump OFF");
}
Buzzer Control: The buzzer is controlled based on gas detection. If gas is detected, the buzzer is activated:
// Control buzzer based on gas detection
if (gasDetected) {
digitalWrite(BUZZER_PIN, HIGH); // Turn on the buzzer
Serial.println("Gas Detected! Buzzer ON");
} else {
digitalWrite(BUZZER_PIN, LOW); // Turn off the buzzer
Serial.println("Gas Not Detected. Buzzer OFF");
}
LCD Display: Sensor readings are displayed on the LCD in a two-step sequence: first, temperature and humidity; second, soil moisture and light status. Each set of data is displayed for 2 seconds:
// Display readings on LCD
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Temp:");
lcd.print(temperature);
lcd.print("C");
lcd.setCursor(0, 1);
lcd.print("Humidity:");
lcd.print(humidity);
lcd.print("%");
delay(2000); // Display first set of data for 2 seconds
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Soil:");
lcd.print(soilMoisturePercent);
lcd.print("%");
lcd.setCursor(0, 1);
lcd.print("Light:");
lcd.print(isDark ? "Dark" : "Light");
delay(2000); // Display second set of data for 2 seconds
Serial Output: Sensor readings and system statuses are printed to the serial monitor for debugging and monitoring purposes:
Serial.print("Soil Moisture: ");
Serial.print(soilMoistureValue);
Serial.print(" (");
Serial.print(soilMoisturePercent);
Serial.println("%)");
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println("C");
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.println("%");
Serial.print("LDR Status: ");
Serial.println(isDark ? "Dark" : "Light");
Serial.print("Gas Status: ");
Serial.println(gasDetected ? "Detected" : "Not Detected");
delay(2000); // Delay for 2 seconds before the next reading
}
Challenges and Solutions
During the development of the IoT-based plant monitoring system, several challenges were encountered. These included sensor calibration issues, connectivity problems, and optimizing the power consumption of the system. Each challenge was addressed by fine-tuning sensor readings, improving the stability of wireless communication, and incorporating efficient power management techniques.
Future Work
There are several potential improvements for the system, such as integrating more advanced sensors for better accuracy, implementing a solar power system for sustainable energy, and enhancing the user app with more features. Additionally, the project can be expanded to monitor and control multiple plants simultaneously.
Conclusion
The IoT-based plant monitoring system successfully automates the watering process and monitors essential environmental parameters to ensure optimal plant health. The system's design and implementation demonstrate the effective integration of hardware and software components to create a reliable and user-friendly solution for plant care.